Build Your AI Web Scraping Agent for Free: Step-by-Step Guide
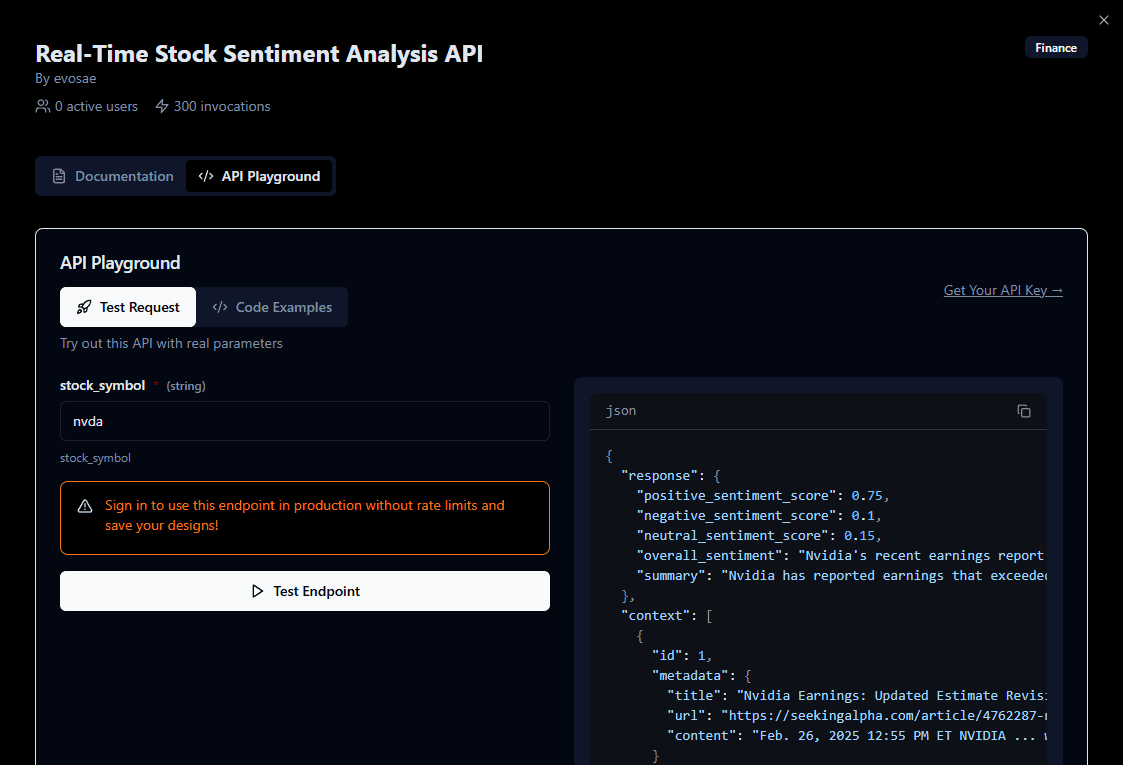
Introduction
Building a web scraping agent has never been easier. With Critique AI, you can create custom API endpoints that use LLMs to intelligently scrape and process web data. This guide walks you through creating your first web scraping agent.
Step-by-Step Guide
1. Design Your API
- Head over to the API studio to begin designing your API
- Define your input parameters
- Specify the output schema
- Configure scraping behavior
2. Test and Iterate
- Use the Critique AI playground to test your API
- Review the results of your API call
- LLMs are not perfect, so you may need to adjust your input parameters or configuration instructions to get the best results.
- Adjust parameters and retry until you're satisfied
3. Publish your API so anyone can use it (and get paid when they do)
- Critique AI's API Marketplace is just beginning to launch, but you can already publish your API and start making money.
- Make money when users with balance on their account use your API.
- Pro tip: APIs that are used for higher-frequency use cases will tend to increase in popularity and usage.
Example Use Case
1// Example published API that can rapidly scrape stock news for sentiment analysis
2// I used this to get an average sentiment score for a stock based on the latest news (ran it like 100 times for a given stock)
3// The url begins with /v1/published-service/ which means it's a published API
4// Notice the data takes in a stock_symbol
5// You can literally try any other code stub for a published API at https://critique-labs.ai/marketplace
6
7function fetchData() {
8 const url = "https://api.critique-labs.ai/v1/published-service/real-time-stock-sentiment-analysis";
9 const data = { "stock_symbol": "string" } ; // replace with actual inputs
10 const headers = {
11 'Content-Type': 'application/json',
12 'X-API-Key': '<YOUR API KEY HERE>'
13 };
14
15 fetch(url, {
16 method: 'POST',
17 headers: headers,
18 body: JSON.stringify(data)
19 })
20 .then(response => response.json())
21 .then(output => {
22 if (output.error) {
23 throw new Error(output.error);
24 }
25 // Output in your specified format
26 const formattedOutput = output.response;
27 // The sources used to generate this output
28 const sources = output.context;
29
30 console.log(formattedOutput);
31 console.log(sources);
32 })
33 .catch(error => {
34 console.error("Error:", error);
35 });
36}
37
38fetchData();
39
You can check out my (beta) polling tool I built to test all marketplace APIs here: https://pollrunner.vercel.app/